说明
使用 chrome.readingList
API 读取和修改阅读清单中的项。
权限
readingList
如需使用 Reading List API,请在扩展程序清单文件中添加 "readingList"
权限:
manifest.json:
{
"name": "My reading list extension",
...
"permissions": [
"readingList"
]
}
可用性
Chrome 的侧边栏中提供了一个阅读清单。让用户可以保存网页以便日后阅读或离线阅读。 使用 Reading List API 检索现有项以及向列表中添加或移除项。
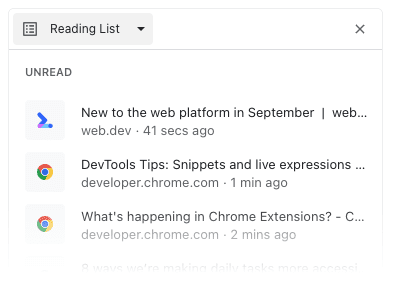
概念和用法
商品排序
阅读清单中的项目不按任何保证顺序排列。
内容唯一性
内容由网址进行键控。这包括哈希和查询字符串。
用例
以下部分演示了 Reading List API 的一些常见用例。如需查看完整的扩展程序示例,请参阅扩展程序示例。
添加项目
如需向阅读清单中添加某项内容,请使用 chrome.readingList.addEntry()
:
chrome.readingList.addEntry({
title: "New to the web platform in September | web.dev",
url: "https://developer.chrome.com/",
hasBeenRead: false
});
显示内容
如需显示阅读清单中的项,请使用 chrome.readingList.query()
方法检索这些项。
const items = await chrome.readingList.query({});
for (const item of items) {
// Do something do display the item
}
将某项内容标记为已读
您可以使用 chrome.readingList.updateEntry()
更新标题、网址和阅读状态。以下代码会将项标记为已读:
chrome.readingList.updateEntry({
url: "https://developer.chrome.com/",
hasBeenRead: true
});
移除项目
如需移除某项内容,请使用 chrome.readingList.removeEntry()
:
chrome.readingList.removeEntry({
url: "https://developer.chrome.com/"
});
扩展程序示例
如需更多 Reading List API 扩展程序演示,请参阅 Reading List API 示例。
类型
AddEntryOptions
属性
-
hasBeenRead
boolean
如果相应条目已被读取,则此值将为
true
。 -
title
string
条目的标题。
-
网址
string
条目的网址。
QueryInfo
属性
-
hasBeenRead
布尔值 选填
指明是搜索已读 (
true
) 项还是未读 (false
) 项。 -
title
字符串(可选)
要搜索的影视内容。
-
网址
字符串(可选)
要搜索的网址。
ReadingListEntry
属性
-
creationTime
number
条目的创建时间。以自 1970 年 1 月 1 日以来的毫秒数记录。
-
hasBeenRead
boolean
如果相应条目已被读取,则此值将为
true
。 -
lastUpdateTime
number
条目的上次更新时间。此值是自 1970 年 1 月 1 日以来的毫秒数。
-
title
string
条目的标题。
-
网址
string
条目的网址。
RemoveOptions
属性
-
网址
string
要移除的网址。
UpdateEntryOptions
属性
-
hasBeenRead
布尔值 选填
更新后的读取状态。如果未提供值,则保留现有状态。
-
title
字符串(可选)
新标题。如果没有提供值,则保留现有图块。
-
网址
string
要更新的网址。
方法
addEntry()
chrome.readingList.addEntry(
entry: AddEntryOptions,
callback?: function,
)
向阅读清单中添加条目(如果相应条目不存在)。
参数
-
要添加到阅读清单中的条目。
-
callback
函数(可选)
callback
参数如下所示:() => void
返回
-
Promise<void>
Manifest V3 及更高版本支持 promise,但提供回调以实现向后兼容性。您不能在同一个函数调用中同时使用这两者。promise 使用传递给回调函数的同一类型进行解析。
query()
chrome.readingList.query(
info: QueryInfo,
callback?: function,
)
检索与 QueryInfo
属性匹配的所有条目。系统不会匹配未提供的属性。
参数
-
资讯
要搜索的属性。
-
callback
函数(可选)
callback
参数如下所示:(entries: ReadingListEntry[]) => void
-
entries
-
返回
-
Promise<ReadingListEntry[]>
Manifest V3 及更高版本支持 promise,但提供回调以实现向后兼容性。您不能在同一个函数调用中同时使用这两者。promise 使用传递给回调函数的同一类型进行解析。
removeEntry()
chrome.readingList.removeEntry(
info: RemoveOptions,
callback?: function,
)
从阅读清单中删除相应条目(如果存在)。
参数
-
要从阅读列表中移除的条目。
-
callback
函数(可选)
callback
参数如下所示:() => void
返回
-
Promise<void>
Manifest V3 及更高版本支持 promise,但提供回调以实现向后兼容性。您不能在同一个函数调用中同时使用这两者。promise 使用传递给回调函数的同一类型进行解析。
updateEntry()
chrome.readingList.updateEntry(
info: UpdateEntryOptions,
callback?: function,
)
更新阅读清单条目(如果存在)。
参数
-
要更新的条目。
-
callback
函数(可选)
callback
参数如下所示:() => void
返回
-
Promise<void>
Manifest V3 及更高版本支持 promise,但提供回调以实现向后兼容性。您不能在同一个函数调用中同时使用这两者。promise 使用传递给回调函数的同一类型进行解析。
活动
onEntryAdded
chrome.readingList.onEntryAdded.addListener(
callback: function,
)
当向阅读清单添加 ReadingListEntry
时触发。
参数
-
callback
功能
callback
参数如下所示:(entry: ReadingListEntry) => void
onEntryRemoved
chrome.readingList.onEntryRemoved.addListener(
callback: function,
)
当从阅读列表中移除 ReadingListEntry
时触发。
参数
-
callback
功能
callback
参数如下所示:(entry: ReadingListEntry) => void
onEntryUpdated
chrome.readingList.onEntryUpdated.addListener(
callback: function,
)
当阅读列表中的 ReadingListEntry
更新时触发。
参数
-
callback
功能
callback
参数如下所示:(entry: ReadingListEntry) => void