说明
使用 chrome.readingList
API 读取和修改阅读列表中的项。
权限
readingList
如需使用 Reading List API,请在扩展程序清单文件中添加 "readingList"
权限:
manifest.json:
{
"name": "My reading list extension",
...
"permissions": [
"readingList"
]
}
可用性
Chrome 的侧边栏上有一个阅读清单。让用户能够保存网页以供日后阅读或离线阅读。 使用 Reading List API 可检索现有项,以及在列表中添加或移除项。
<ph type="x-smartling-placeholder">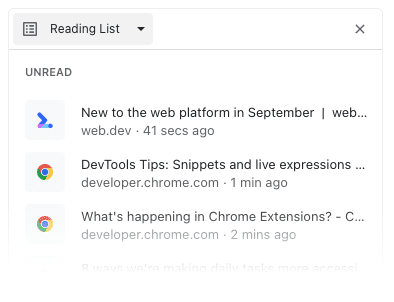
概念和用法
商品排序
阅读清单中的内容没有任何保证顺序。
商品唯一性
各项由网址进行键控。其中包括哈希和查询字符串。
使用场景
以下部分展示了 Reading List API 的一些常见用例。如需查看完整的扩展程序示例,请参阅扩展程序示例。
添加项目
如需将某一项添加到阅读清单中,请使用 chrome.readingList.addEntry()
:
chrome.readingList.addEntry({
title: "New to the web platform in September | web.dev",
url: "https://developer.chrome.com/",
hasBeenRead: false
});
显示项
如需显示阅读列表中的项,请使用 chrome.readingList.query()
方法检索这些项。
方法。
const items = await chrome.readingList.query({});
for (const item of items) {
// Do something do display the item
}
将内容标记为已读
您可以使用 chrome.readingList.updateEntry()
更新标题、网址和阅读状态。以下代码可将某个项标记为已读:
chrome.readingList.updateEntry({
url: "https://developer.chrome.com/",
hasBeenRead: true
});
移除项目
如需移除某项内容,请使用 chrome.readingList.removeEntry()
:
chrome.readingList.removeEntry({
url: "https://developer.chrome.com/"
});
扩展程序示例
如需查看 Reading List API 扩展程序演示,请参阅 Reading List API 示例。
类型
AddEntryOptions
属性
-
hasBeenRead
布尔值
如果条目已被读取,其值将为
true
。 -
标题
字符串
条目的标题。
-
网址
字符串
条目的网址。
QueryInfo
属性
-
hasBeenRead
布尔值(可选)
指明是搜索已读 (
true
) 还是未读 (false
) 内容。 -
标题
字符串(可选)
要搜索的标题。
-
网址
字符串(可选)
要搜索的网址。
ReadingListEntry
属性
-
creationTime
number
条目的创建时间。从 1970 年 1 月 1 日开始记录(以毫秒为单位)。
-
hasBeenRead
布尔值
如果条目已被读取,其值将为
true
。 -
lastUpdateTime
number
上次更新条目的时间。此值是自 1970 年 1 月 1 日以来的毫秒数。
-
标题
字符串
条目的标题。
-
网址
字符串
条目的网址。
RemoveOptions
属性
-
网址
字符串
要移除的网址。
UpdateEntryOptions
属性
-
hasBeenRead
布尔值(可选)
更新的读取状态。如果未提供值,则现有状态会保留下来。
-
标题
字符串(可选)
新标题。如果未提供值,则现有图块会保留。
-
网址
字符串
要更新的网址。
方法
addEntry()
chrome.readingList.addEntry(
entry: AddEntryOptions,
callback?: function,
)
在阅读清单中添加一个不存在的条目。
参数
-
要添加到阅读清单的条目。
-
callback
函数(可选)
callback
参数如下所示:() => void
返回
-
承诺<void>
Manifest V3 及更高版本支持 Promise,但为以下项目提供回调: 向后兼容性您不能在同一个函数调用中同时使用这两者。通过 promise 使用传递给回调的类型进行解析。
query()
chrome.readingList.query(
info: QueryInfo,
callback?: function,
)
检索与 QueryInfo
属性匹配的所有条目。未提供的属性将不匹配。
参数
-
资讯
要搜索的属性。
-
callback
函数(可选)
callback
参数如下所示:(entries: ReadingListEntry[]) => void
-
entries
-
返回
-
Promise<ReadingListEntry[]>
Manifest V3 及更高版本支持 Promise,但为以下项目提供回调: 向后兼容性您不能在同一个函数调用中同时使用这两者。通过 promise 使用传递给回调的类型进行解析。
removeEntry()
chrome.readingList.removeEntry(
info: RemoveOptions,
callback?: function,
)
从阅读清单中删除条目(如果存在)。
参数
-
要从阅读清单中移除的条目。
-
callback
函数(可选)
callback
参数如下所示:() => void
返回
-
承诺<void>
Manifest V3 及更高版本支持 Promise,但为以下项目提供回调: 向后兼容性您不能在同一个函数调用中同时使用这两者。通过 promise 使用传递给回调的类型进行解析。
updateEntry()
chrome.readingList.updateEntry(
info: UpdateEntryOptions,
callback?: function,
)
更新阅读清单条目(如果存在)。
参数
-
要更新的条目。
-
callback
函数(可选)
callback
参数如下所示:() => void
返回
-
承诺<void>
Manifest V3 及更高版本支持 Promise,但为以下项目提供回调: 向后兼容性您不能在同一个函数调用中同时使用这两者。通过 promise 使用传递给回调的类型进行解析。
事件
onEntryAdded
chrome.readingList.onEntryAdded.addListener(
callback: function,
)
当有 ReadingListEntry
被添加到阅读清单时触发。
参数
-
callback
函数
callback
参数如下所示:(entry: ReadingListEntry) => void
onEntryRemoved
chrome.readingList.onEntryRemoved.addListener(
callback: function,
)
当 ReadingListEntry
从阅读清单中移除时触发。
参数
-
callback
函数
callback
参数如下所示:(entry: ReadingListEntry) => void
onEntryUpdated
chrome.readingList.onEntryUpdated.addListener(
callback: function,
)
当读取列表中的某个 ReadingListEntry
更新时触发。
参数
-
callback
函数
callback
参数如下所示:(entry: ReadingListEntry) => void