如同擴充功能可讓使用者自訂 Chrome 瀏覽器一樣,選項頁面也會啟用 自訂擴充功能透過選項啟用功能並允許使用者選擇 瞭解他們需要哪些功能
找到選項頁面
使用者可以透過「直接連結」存取選項頁面,也可以在工具列的擴充功能圖示上按一下滑鼠右鍵,然後選取選項。此外,使用者也可以先開啟 chrome://extensions
,找出想要的擴充功能,然後按一下「詳細資料」,再選取選項連結,就可以前往選項頁面。
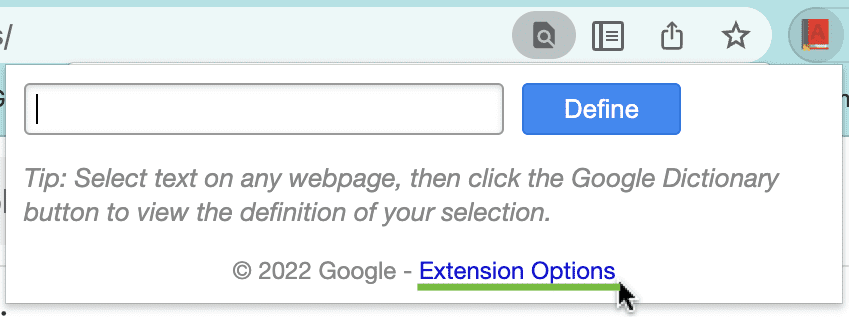
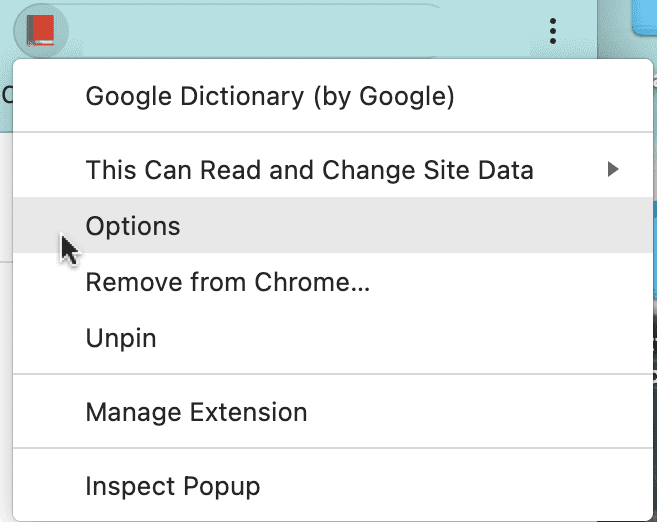
撰寫選項頁面
以下是選項頁面範例:
options.html:
<!DOCTYPE html>
<html>
<head>
<title>My Test Extension Options</title>
</head>
<body>
<select id="color">
<option value="red">red</option>
<option value="green">green</option>
<option value="blue">blue</option>
<option value="yellow">yellow</option>
</select>
<label>
<input type="checkbox" id="like" />
I like colors.
</label>
<div id="status"></div>
<button id="save">Save</button>
<script src="options.js"></script>
</body>
</html>
以下是選項指令碼範例。將其儲存在與 options.html
相同的資料夾中。
這項設定會透過 storage.sync
API,在所有裝置上儲存使用者偏好的選項。
options.js:
// Saves options to chrome.storage
const saveOptions = () => {
const color = document.getElementById('color').value;
const likesColor = document.getElementById('like').checked;
chrome.storage.sync.set(
{ favoriteColor: color, likesColor: likesColor },
() => {
// Update status to let user know options were saved.
const status = document.getElementById('status');
status.textContent = 'Options saved.';
setTimeout(() => {
status.textContent = '';
}, 750);
}
);
};
// Restores select box and checkbox state using the preferences
// stored in chrome.storage.
const restoreOptions = () => {
chrome.storage.sync.get(
{ favoriteColor: 'red', likesColor: true },
(items) => {
document.getElementById('color').value = items.favoriteColor;
document.getElementById('like').checked = items.likesColor;
}
);
};
document.addEventListener('DOMContentLoaded', restoreOptions);
document.getElementById('save').addEventListener('click', saveOptions);
最後,將 "storage"
權限新增至擴充功能的資訊清單檔案:
manifest.json:
{
"name": "My extension",
...
"permissions": [
"storage"
]
...
}
宣告選項頁面行為
擴充功能選項頁面有兩種,分別是完整頁面和內嵌。類型 選項頁面的組成方式取決於資訊清單中的宣告方式
完整頁面選項
「完整頁面」選項頁面會顯示在新分頁中。在資訊清單的 "options_page"
欄位中註冊選項 HTML 檔案。
manifest.json:
{
"name": "My extension",
...
"options_page": "options.html",
...
}
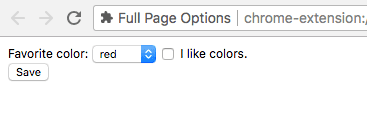
內嵌選項
內嵌選項頁面可讓使用者調整擴充功能選項,不必離開
內嵌方塊中的擴充功能管理頁面如要宣告嵌入選項,請註冊 HTML
檔案,並將 "open_in_tab"
鍵設為 false
。"options_ui"
manifest.json:
{
"name": "My extension",
...
"options_ui": {
"page": "options.html",
"open_in_tab": false
},
...
}
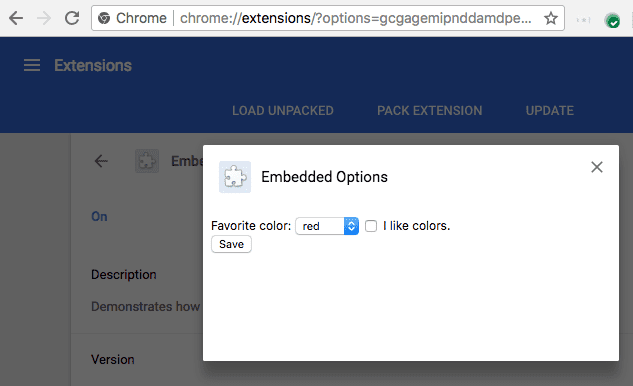
page
(字串)- 指定選項頁面相對於擴充功能根層級的路徑。
open_in_tab
(布林值)- 指出擴充功能的選項頁面是否會在新分頁中開啟。如果設為
false
,擴充功能的選項頁面會嵌入chrome://extensions
中,而非在新分頁中開啟。
考量差異
內嵌於 chrome://extensions
中的選項頁面與分頁中選項頁面的行為有些微差異。
選項頁面的連結
擴充功能可以呼叫
chrome.runtime.openOptionsPage()
。舉例來說,你可以加入彈出式視窗:
popup.html:
<button id="go-to-options">Go to options</button>
<script src="popup.js"></script>
popup.js:
document.querySelector('#go-to-options').addEventListener('click', function() {
if (chrome.runtime.openOptionsPage) {
chrome.runtime.openOptionsPage();
} else {
window.open(chrome.runtime.getURL('options.html'));
}
});
分頁 API
由於內嵌選項程式碼並非由分頁代管,因此無法使用 Tabs API。
請改用 runtime.connect()
和 runtime.sendMessage()
,
表示選項頁面需要操控包含的分頁。
通訊 API
如果擴充功能的選項頁面使用 runtime.connect()
或
runtime.sendMessage()
,系統不會設定寄件者的分頁,且寄件者的網址
設為選項頁面的網址
尺寸
內嵌選項應根據頁面內容自動決定尺寸。不過 就某些類型的內容而言,內嵌框可能就沒有適當大小。這個問題最常見 可根據視窗大小調整內容形狀的選項頁面。
如果這是問題,請在選項頁面提供固定的尺寸,以確保 就會找到適合的尺寸