タイトルがひどいのでごめんなさい。でも残念でした。
Chrome 44 では、Notfication.data と ServiceWorkerRegistration.getNotifications() が追加され、プッシュ メッセージで通知を処理する際の一般的なユースケースが拡張 / 簡素化されました。
通知データ
Notification.data を使用すると、JavaScript オブジェクトを Notification に関連付けることができます。
つまり、プッシュ メッセージを受信したときに、データを含む通知を作成できます。その後、notificationclick イベントで、クリックされた通知を取得してそのデータを取得できます。
たとえば、次のようにデータ オブジェクトを作成して通知オプションに追加します。
self.addEventListener('push', function(event) {
console.log('Received a push message', event);
var title = 'Yay a message.';
var body = 'We have received a push message.';
var icon = '/images/icon-192x192.png';
var tag = 'simple-push-demo-notification-tag';
var data = {
doge: {
wow: 'such amaze notification data'
}
};
event.waitUntil(
self.registration.showNotification(title, {
body: body,
icon: icon,
tag: tag,
data: data
})
);
});
つまり、notificationclick イベントで情報を取得できます。
self.addEventListener('notificationclick', function(event) {
var doge = event.notification.data.doge;
console.log(doge.wow);
});
これまでは、データを IndexDB に保存するか、アイコンの URL の末尾に何かを追加する必要がありました。
ServiceWorkerRegistration.getNotifications()
プッシュ通知を扱うデベロッパーからよく寄せられるリクエストの 1 つは、表示される通知をより細かく制御できるようにすることです。
たとえば、ユーザーが複数のメッセージを送信し、受信者に複数の通知が表示されるチャット アプリケーションがユースケースとして考えられます。理想的には、ウェブアプリは、表示されていない通知が複数あることを認識し、それらを 1 つの通知にまとめられるようにします。
getNotifications() を使用しない場合は、以前の通知を最新のメッセージに置き換えることをおすすめします。getNotifications() を使用すると、通知がすでに表示されている場合は通知を「閉じる」ことができ、ユーザー エクスペリエンスが大幅に向上します。
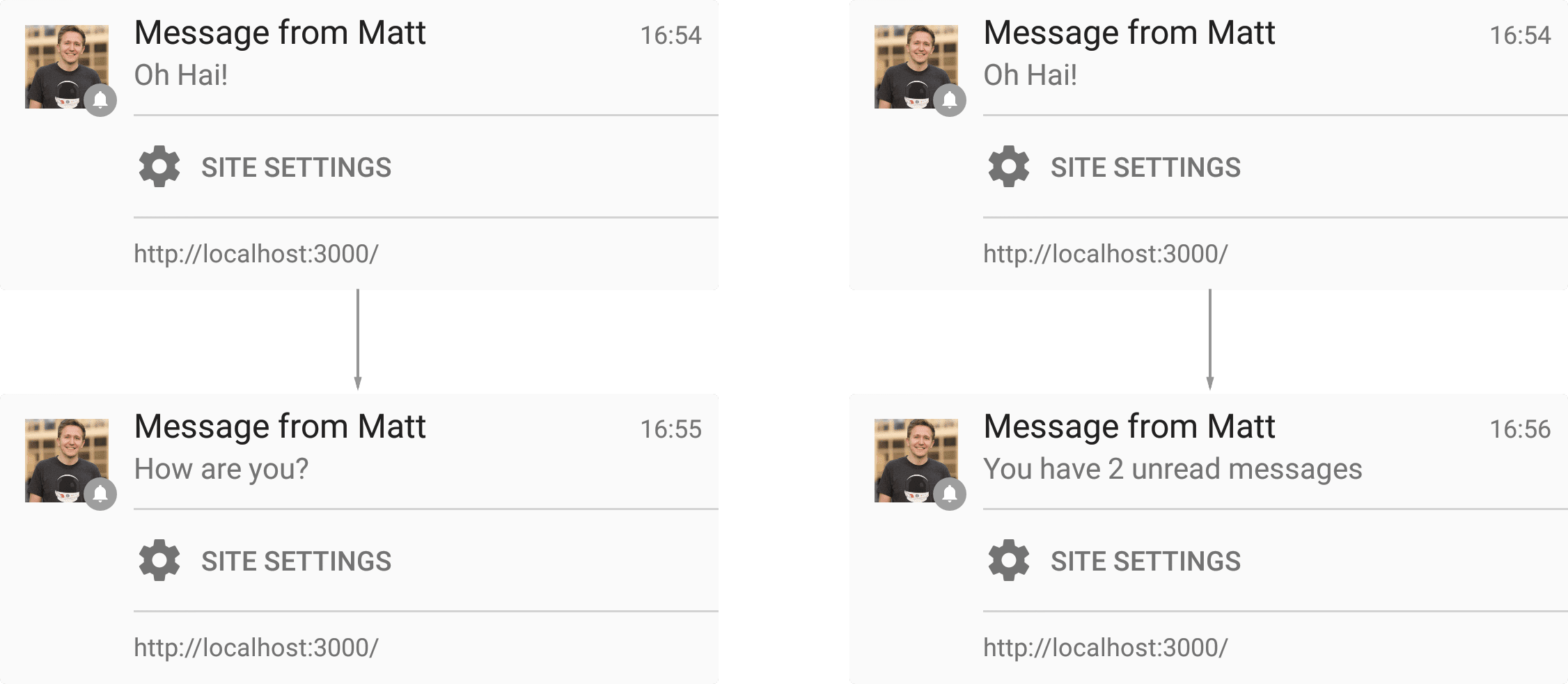
そのためのコードは比較的シンプルです。プッシュ イベント内で ServiceWorkerRegistration.getNotifications() を呼び出して、現在の通知の配列を取得し、そこから適切な動作(すべての通知を閉じるか、Notification.tag を使用するか)を決定します。
function showNotification(title, body, icon, data) {
var notificationOptions = {
body: body,
icon: icon ? icon : 'images/touch/chrome-touch-icon-192x192.png',
tag: 'simple-push-demo-notification',
data: data
};
self.registration.showNotification(title, notificationOptions);
return;
}
self.addEventListener('push', function(event) {
console.log('Received a push message', event);
// Since this is no payload data with the first version
// of Push notifications, here we'll grab some data from
// an API and use it to populate a notification
event.waitUntil(
fetch(API_ENDPOINT).then(function(response) {
if (response.status !== 200) {
console.log('Looks like there was a problem. Status Code: ' +
response.status);
// Throw an error so the promise is rejected and catch() is executed
throw new Error();
}
// Examine the text in the response
return response.json().then(function(data) {
var title = 'You have a new message';
var message = data.message;
var icon = 'images/notification-icon.png';
var notificationTag = 'chat-message';
var notificationFilter = {
tag: notificationTag
};
return self.registration.getNotifications(notificationFilter)
.then(function(notifications) {
if (notifications && notifications.length > 0) {
// Start with one to account for the new notification
// we are adding
var notificationCount = 1;
for (var i = 0; i < notifications.length; i++) {
var existingNotification = notifications[i];
if (existingNotification.data &&
existingNotification.data.notificationCount) {
notificationCount +=
existingNotification.data.notificationCount;
} else {
notificationCount++;
}
existingNotification.close();
}
message = 'You have ' + notificationCount +
' weather updates.';
notificationData.notificationCount = notificationCount;
}
return showNotification(title, message, icon, notificationData);
});
});
}).catch(function(err) {
console.error('Unable to retrieve data', err);
var title = 'An error occurred';
var message = 'We were unable to get the information for this ' +
'push message';
return showNotification(title, message);
})
);
});
self.addEventListener('notificationclick', function(event) {
console.log('On notification click: ', event);
if (Notification.prototype.hasOwnProperty('data')) {
console.log('Using Data');
var url = event.notification.data.url;
event.waitUntil(clients.openWindow(url));
} else {
event.waitUntil(getIdb().get(KEY_VALUE_STORE_NAME,
event.notification.tag).then(function(url) {
// At the moment you cannot open third party URL's, a simple trick
// is to redirect to the desired URL from a URL on your domain
var redirectUrl = '/redirect.html?redirect=' +
url;
return clients.openWindow(redirectUrl);
}));
}
});
このコードスニペットでまず注目すべき点は、getNotifications() にフィルタ オブジェクトを渡すことで通知をフィルタすることです。つまり、特定のタグ(この例では特定の会話)の通知のリストを取得できます。
var notificationFilter = {
tag: notificationTag
};
return self.registration.getNotifications(notificationFilter)
次に、表示されている通知を確認し、その通知に関連付けられている通知数があるかどうかを確認して、その数に基づいてインクリメントします。たとえば、未読のメッセージが 2 件あることをユーザーに伝える通知が 1 件ある場合、新しいプッシュが届いたときに未読のメッセージが 3 件あることをユーザーに伝えます。
var notificationCount = 1;
for (var i = 0; i < notifications.length; i++) {
var existingNotification = notifications[i];
if (existingNotification.data && existingNotification.data.notificationCount) {
notificationCount += existingNotification.data.notificationCount;
} else {
notificationCount++;
}
existingNotification.close();
}
重要な点として、通知で close()
を呼び出して、通知が通知リストから削除されるようにする必要があります。これは Chrome のバグです。同じタグが使用されているため、各通知が次の通知に置き換えられます。現時点では、この置換は getNotifications()
から返される配列には反映されません。
これは getNotifications() の一例にすぎず、ご想像のとおり、この API は他にもさまざまなユースケースを開くことができます。
NotificationOptions.vibrate
Chrome 45 以降では、通知を作成するときにバイブレーション パターンを指定できます。Vibration API に対応しているデバイス(現在は Chrome for Android のみ)では、通知が表示されるときのバイブレーション パターンをカスタマイズできます。
バイブレーション パターンは、数値の配列か、1 つの数値の配列として扱われる単一の数値のいずれかです。配列内の値はミリ秒単位の時間で表され、偶数のインデックス(0、2、4、...)は振動する時間、奇数のインデックスは、次の振動までの一時停止時間です。
self.registration.showNotification('Buzz!', {
body: 'Bzzz bzzzz',
vibrate: [300, 100, 400] // Vibrate 300ms, pause 100ms, then vibrate 400ms
});
その他の一般的な機能リクエスト
開発者からよく寄せられる要望の一つは、一定期間後に通知を閉じる機能、または通知が表示されている場合は閉じるだけを目的としてプッシュ通知を送信する機能です。
現時点では、この方法で設定する方法はなく、仕様でも許可されていません。Chrome エンジニアリング チームはこのユースケースを認識しています。
Android 通知
パソコンでは、次のコードを使用して通知を作成できます。
new Notification('Hello', {body: 'Yay!'});
これは、プラットフォームの制限により Android ではサポートされていません。具体的には、Chrome は onclick などの Notification オブジェクトのコールバックをサポートできません。ただし、パソコンでは、現在開いているウェブアプリの通知を表示するために使用されます。
言及する理由は、元々は、以下のようなシンプルな機能検出で、デスクトップをサポートし、Android でエラーが発生しないようにできていたことです。
if (!'Notification' in window) {
// Notifications aren't supported
return;
}
ただし、Android 版 Chrome でプッシュ通知がサポートされたため、通知は ServiceWorker から作成できますが、ウェブページからは作成できなくなりました。つまり、この機能検出は適切ではなくなりました。Android 版 Chrome で通知を作成しようとすると、次のエラー メッセージが表示されます。
_Uncaught TypeError: Failed to construct 'Notification': Illegal constructor.
Use ServiceWorkerRegistration.showNotification() instead_
現時点で Android とパソコンの機能検出を行う最善の方法は次のとおりです。
function isNewNotificationSupported() {
if (!window.Notification || !Notification.requestPermission)
return false;
if (Notification.permission == 'granted')
throw new Error('You must only call this \*before\* calling
Notification.requestPermission(), otherwise this feature detect would bug the
user with an actual notification!');
try {
new Notification('');
} catch (e) {
if (e.name == 'TypeError')
return false;
}
return true;
}
これは次のように使用できます。
if (window.Notification && Notification.permission == 'granted') {
// We would only have prompted the user for permission if new
// Notification was supported (see below), so assume it is supported.
doStuffThatUsesNewNotification();
} else if (isNewNotificationSupported()) {
// new Notification is supported, so prompt the user for permission.
showOptInUIForNotifications();
}