简介
媒体来源扩展 (MSE) 为 HTML5 <audio>
和 <video>
元素提供扩展的缓冲和播放控件。虽然最初的开发目的是支持基于基于 HTTP 的动态自适应流式传输 (DASH) 的视频播放器,但我们将在下文介绍如何将其用于音频:专门用于无间断播放。
你可能在听过一张音乐专辑,其中的歌曲会流畅地穿梭于不同的曲目中;你甚至可能正在听一首歌。音乐人打造了这种无间断播放体验,既是一种艺术选择,也是一种黑胶唱片和 CD 的制品,其中音频是以连续串流形式录制的。遗憾的是,受 MP3 和 AAC 等现代音频编解码器的运作方式影响,这种流畅的听觉体验在当今经常无法成功。
我们将在下文中详细了解具体原因,但现在我们先进行演示。下面是出色的 Sintel 视频的前 30 秒,拆分成五个单独的 MP3 文件并使用 MSE 重新组合。红线表示每个 MP3 的创建(编码)过程中引入的间隙;在这些时间点,你会听到一些故障
糟糕!这种体验可能不太好;我们可以做得更好。再多做一点工作,使用上面演示中完全相同的 MP3 文件,我们就可以使用 MSE 消除这些烦人的缺口。下一个演示中的绿线表示文件的连接位置和间隙已去除。如果使用的是 Chrome 38 及更高版本,则可以无缝播放!
制作无间断内容的方法有多种。在本演示中,我们将重点关注普通用户可能会随意放置的文件类型。每个文件都单独进行了编码,而不考虑其前后的音频片段。
基本设置
首先,让我们回顾一下并介绍 MediaSource
实例的基本设置。顾名思义,媒体来源扩展程序只是现有媒体元素的扩展。在下面,我们为音频元素的来源属性分配了代表 MediaSource
实例的 Object URL
;就像设置标准网址一样
var audio = document.createElement('audio');
var mediaSource = new MediaSource();
var SEGMENTS = 5;
mediaSource.addEventListener('sourceopen', function() {
var sourceBuffer = mediaSource.addSourceBuffer('audio/mpeg');
function onAudioLoaded(data, index) {
// Append the ArrayBuffer data into our new SourceBuffer.
sourceBuffer.appendBuffer(data);
}
// Retrieve an audio segment via XHR. For simplicity, we're retrieving the
// entire segment at once, but we could also retrieve it in chunks and append
// each chunk separately. MSE will take care of assembling the pieces.
GET('sintel/sintel_0.mp3', function(data) { onAudioLoaded(data, 0); } );
});
audio.src = URL.createObjectURL(mediaSource);
连接 MediaSource
对象后,它会执行一些初始化并最终触发 sourceopen
事件;此时,我们可以创建一个 SourceBuffer
。在上面的示例中,我们创建了 audio/mpeg
,它能够解析和解码 MP3 片段;还有几种其他类型可供选择。
异常波形
我们稍后会返回到代码,但现在我们来详细了解一下刚刚附加的文件,特别是在文件末尾。下图显示了来自 sintel_0.mp3
轨道的最近 3, 000 个样本在两个渠道的平均值。红线中的每个像素都是 [-1.0, 1.0]
范围内的一个浮点样本。
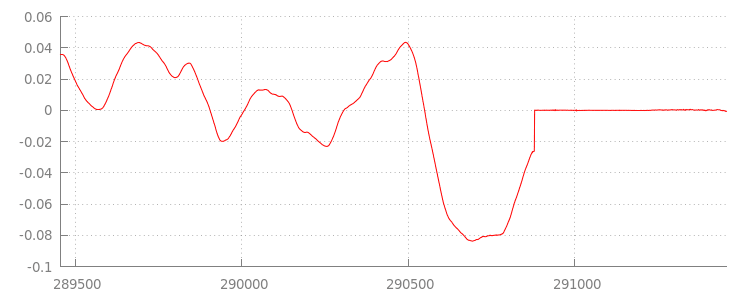
那些零(无声)样本是怎么回事?它们实际上是由编码期间引入的压缩失真造成的。几乎每个编码器都会引入某种类型的填充。在本例中,LAME 在文件末尾正好添加了 576 个填充样本。
除了末尾的内边距之外,每个文件的开头部分也添加了内边距。如果我们向前看 sintel_1.mp3
轨道,会看到前面还有 576 个内边距样本。内边距因编码器和内容而异,但我们会根据每个文件中包含的 metadata
知道确切的值。
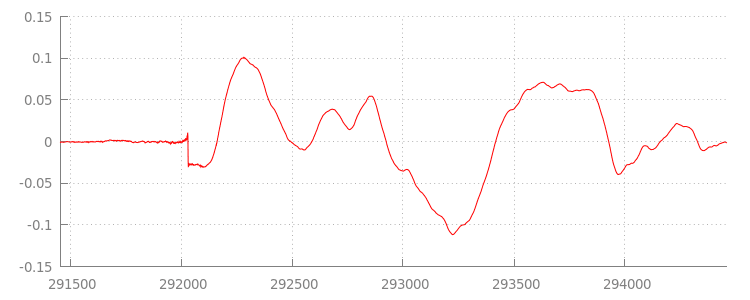
在上一个演示中,每个文件开头和结尾的无声部分是导致片段之间出现“错误”的原因。为了实现无间断播放,我们需要移除这些无声部分。幸运的是,使用 MediaSource
可以轻松做到这一点。下面,我们将修改 onAudioLoaded()
方法,以使用附加窗口和时间戳偏移来移除此无声部分。
示例代码
function onAudioLoaded(data, index) {
// Parsing gapless metadata is unfortunately non trivial and a bit messy, so
// we'll glaze over it here; see the appendix for details.
// ParseGaplessData() will return a dictionary with two elements:
//
// audioDuration: Duration in seconds of all non-padding audio.
// frontPaddingDuration: Duration in seconds of the front padding.
//
var gaplessMetadata = ParseGaplessData(data);
// Each appended segment must be appended relative to the next. To avoid any
// overlaps, we'll use the end timestamp of the last append as the starting
// point for our next append or zero if we haven't appended anything yet.
var appendTime = index > 0 ? sourceBuffer.buffered.end(0) : 0;
// Simply put, an append window allows you to trim off audio (or video) frames
// which fall outside of a specified time range. Here, we'll use the end of
// our last append as the start of our append window and the end of the real
// audio data for this segment as the end of our append window.
sourceBuffer.appendWindowStart = appendTime;
sourceBuffer.appendWindowEnd = appendTime + gaplessMetadata.audioDuration;
// The timestampOffset field essentially tells MediaSource where in the media
// timeline the data given to appendBuffer() should be placed. I.e., if the
// timestampOffset is 1 second, the appended data will start 1 second into
// playback.
//
// MediaSource requires that the media timeline starts from time zero, so we
// need to ensure that the data left after filtering by the append window
// starts at time zero. We'll do this by shifting all of the padding we want
// to discard before our append time (and thus, before our append window).
sourceBuffer.timestampOffset =
appendTime - gaplessMetadata.frontPaddingDuration;
// When appendBuffer() completes, it will fire an updateend event signaling
// that it's okay to append another segment of media. Here, we'll chain the
// append for the next segment to the completion of our current append.
if (index == 0) {
sourceBuffer.addEventListener('updateend', function() {
if (++index < SEGMENTS) {
GET('sintel/sintel_' + index + '.mp3',
function(data) { onAudioLoaded(data, index); });
} else {
// We've loaded all available segments, so tell MediaSource there are no
// more buffers which will be appended.
mediaSource.endOfStream();
URL.revokeObjectURL(audio.src);
}
});
}
// appendBuffer() will now use the timestamp offset and append window settings
// to filter and timestamp the data we're appending.
//
// Note: While this demo uses very little memory, more complex use cases need
// to be careful about memory usage or garbage collection may remove ranges of
// media in unexpected places.
sourceBuffer.appendBuffer(data);
}
无缝的波形
让我们在应用附加窗口后再次查看波形,看看我们全新的代码都取得了哪些成就。如下所示,sintel_0.mp3
末尾的静音部分(红色)和 sintel_1.mp3
开头的静音部分(蓝色)已被移除;能够在细分受众群之间无缝切换
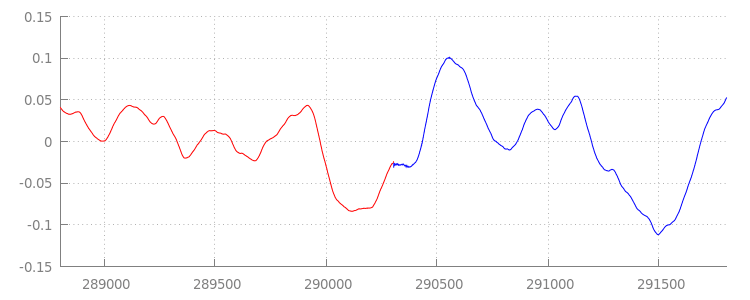
总结
至此,我们已经将所有五个细分无缝拼接成了一个视频,演示到此结束。开始之前,您可能已经注意到,onAudioLoaded()
方法没有考虑容器或编解码器。这意味着,无论容器或编解码器类型如何,所有这些技术都适用。您可以在下方重放原始演示版 DASH-ready Fragment MP4(而非 MP3)。
如需了解更多信息,请查看下面的附录,深入了解无间断的内容创建和元数据解析。您也可以探索 gapless.js
,进一步了解支持此演示的代码。
感谢您阅读本邮件!
附录 A:制作无间断的内容
制作无间断的内容可能并非易事。下面,我们将介绍如何创建此演示中使用的 Sintel 媒体。首先,您需要一份 Sintel 的无损 FLAC 音轨的副本;,则 SHA1 如下所示。对于工具,您需要 FFmpeg、MP4Box、LAME,并使用 afconvert 在 OSX 上安装。
unzip Jan_Morgenstern-Sintel-FLAC.zip
sha1sum 1-Snow_Fight.flac
# 0535ca207ccba70d538f7324916a3f1a3d550194 1-Snow_Fight.flac
首先,我们将拆分 1-Snow_Fight.flac
轨道的前 31.5 秒。我们还想添加一个从 28 秒处开始的 2.5 秒淡出,以避免在播放结束后产生任何点击。使用下面的 FFmpeg 命令行,我们可以完成所有这些操作,并将结果放在 sintel.flac
中。
ffmpeg -i 1-Snow_Fight.flac -t 31.5 -af "afade=t=out:st=28:d=2.5" sintel.flac
接下来,我们将该文件拆分为 5 个 wave 文件,每个文件 6.5 秒;使用 wave 最为简单,因为几乎所有编码器都支持提取 wave。同样,我们可以使用 FFmpeg 来精确执行此操作,之后将得到 sintel_0.wav
、sintel_1.wav
、sintel_2.wav
、sintel_3.wav
和 sintel_4.wav
。
ffmpeg -i sintel.flac -acodec pcm_f32le -map 0 -f segment \
-segment_list out.list -segment_time 6.5 sintel_%d.wav
接下来,我们来创建 MP3 文件LAME 提供了多种创建无间断内容的选项。如果您能控制内容,不妨考虑使用 --nogap
对所有文件进行批量编码,以避免片段之间完全填充。但在本演示中,我们想要该内边距,因此我们将使用 wave 文件的标准高质量 VBR 编码。
lame -V=2 sintel_0.wav sintel_0.mp3
lame -V=2 sintel_1.wav sintel_1.mp3
lame -V=2 sintel_2.wav sintel_2.mp3
lame -V=2 sintel_3.wav sintel_3.mp3
lame -V=2 sintel_4.wav sintel_4.mp3
以上就是创建 MP3 文件所需的所有内容。现在,我们将介绍如何创建碎片化的 MP4 文件。我们将按照 Apple 的说明创建适用于 iTunes 的媒体。在下文中,我们会先按照说明将 wave 文件转换为中间的 CAF 文件,然后再使用推荐的参数在 MP4 容器中将其编码为 AAC 文件。
afconvert sintel_0.wav sintel_0_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_1.wav sintel_1_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_2.wav sintel_2_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_3.wav sintel_3_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_4.wav sintel_4_intermediate.caf -d 0 -f caff \
--soundcheck-generate
afconvert sintel_0_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_0.m4a
afconvert sintel_1_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_1.m4a
afconvert sintel_2_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_2.m4a
afconvert sintel_3_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_3.m4a
afconvert sintel_4_intermediate.caf -d aac -f m4af -u pgcm 2 --soundcheck-read \
-b 256000 -q 127 -s 2 sintel_4.m4a
现在,我们有几个 M4A 文件,我们需要对这些文件进行适当碎片化,然后才能将其与 MediaSource
一起使用。出于我们的目的,我们将使用大小为 1 秒的 fragment。MP4Box 会将每个碎片化的 MP4 作为 sintel_#_dashinit.mp4
以及可舍弃的 MPEG-DASH 清单 (sintel_#_dash.mpd
) 写出。
MP4Box -dash 1000 sintel_0.m4a && mv sintel_0_dashinit.mp4 sintel_0.mp4
MP4Box -dash 1000 sintel_1.m4a && mv sintel_1_dashinit.mp4 sintel_1.mp4
MP4Box -dash 1000 sintel_2.m4a && mv sintel_2_dashinit.mp4 sintel_2.mp4
MP4Box -dash 1000 sintel_3.m4a && mv sintel_3_dashinit.mp4 sintel_3.mp4
MP4Box -dash 1000 sintel_4.m4a && mv sintel_4_dashinit.mp4 sintel_4.mp4
rm sintel_{0,1,2,3,4}_dash.mpd
大功告成!我们现在拥有碎片化的 MP4 和 MP3 文件,其中包含进行无间断播放所需的正确元数据。请参阅附录 B,详细了解元数据是什么样子的。
附录 B:解析无间断元数据
就像创建无间断的内容一样,解析无间断元数据可能会很棘手,因为没有标准的存储方法。下面,我们将介绍两种最常见的编码器(LAME 和 iTunes)如何存储其无间断元数据。首先,为上面使用的 ParseGaplessData()
设置一些辅助方法和大纲。
// Since most MP3 encoders store the gapless metadata in binary, we'll need a
// method for turning bytes into integers. Note: This doesn't work for values
// larger than 2^30 since we'll overflow the signed integer type when shifting.
function ReadInt(buffer) {
var result = buffer.charCodeAt(0);
for (var i = 1; i < buffer.length; ++i) {
result <<../= 8;
result += buffer.charCodeAt(i);
}
return result;
}
function ParseGaplessData(arrayBuffer) {
// Gapless data is generally within the first 512 bytes, so limit parsing.
var byteStr = new TextDecoder().decode(arrayBuffer.slice(0, 512));
var frontPadding = 0, endPadding = 0, realSamples = 0;
// ... we'll fill this in as we go below.
我们首先介绍 Apple 的 iTunes 元数据格式,因为它最容易解析和解释。在 MP3 和 M4A 文件中,iTunes(和 afconvert)可以用 ASCII 书写一个短小段,如下所示:
iTunSMPB[ 26 bytes ]0000000 00000840 000001C0 0000000000046E00
该代码位于 MP3 容器内的 ID3 标记内和 MP4 容器内的元数据 Atom 中。出于我们的目的,我们可以忽略第一个 0000000
令牌。接下来的三个词元是前填充、结束填充和非填充样本总数。用每个音频的采样率除以音频的采样率,就得出了每个音频的时长。
// iTunes encodes the gapless data as hex strings like so:
//
// 'iTunSMPB[ 26 bytes ]0000000 00000840 000001C0 0000000000046E00'
// 'iTunSMPB[ 26 bytes ]####### frontpad endpad real samples'
//
// The approach here elides the complexity of actually parsing MP4 atoms. It
// may not work for all files without some tweaks.
var iTunesDataIndex = byteStr.indexOf('iTunSMPB');
if (iTunesDataIndex != -1) {
var frontPaddingIndex = iTunesDataIndex + 34;
frontPadding = parseInt(byteStr.substr(frontPaddingIndex, 8), 16);
var endPaddingIndex = frontPaddingIndex + 9;
endPadding = parseInt(byteStr.substr(endPaddingIndex, 8), 16);
var sampleCountIndex = endPaddingIndex + 9;
realSamples = parseInt(byteStr.substr(sampleCountIndex, 16), 16);
}
另一方面,大多数开源 MP3 编码器会将无间断元数据存储在一个特殊的 Xing 标头中,该标头位于一个静默 MPEG 帧内(该标头是静默的,所以不了解 Xing 标头的解码器只会播放静音)。遗憾的是,该标记并非始终存在,并且包含许多可选字段。在此演示中,我们可以控制媒体,但在实践中,需要执行一些额外的检查才能知道无间断元数据实际可用的时间。
首先,我们将解析总样本数。为简单起见,我们将从 Xing 标头读取此内容,不过,您也可以通过常规的 MPEG 音频标头构建它。Xing 标头可以使用 Xing
或 Info
标记进行标记。在此标记之后正好 4 个字节,有 32 位表示文件中的总帧数;将此值乘以每帧的样本数即可得到文件中的样本总数。
// Xing padding is encoded as 24bits within the header. Note: This code will
// only work for Layer3 Version 1 and Layer2 MP3 files with XING frame counts
// and gapless information. See the following document for more details:
// http://www.codeproject.com/Articles/8295/MPEG-Audio-Frame-Header
var xingDataIndex = byteStr.indexOf('Xing');
if (xingDataIndex == -1) xingDataIndex = byteStr.indexOf('Info');
if (xingDataIndex != -1) {
// See section 2.3.1 in the link above for the specifics on parsing the Xing
// frame count.
var frameCountIndex = xingDataIndex + 8;
var frameCount = ReadInt(byteStr.substr(frameCountIndex, 4));
// For Layer3 Version 1 and Layer2 there are 1152 samples per frame. See
// section 2.1.5 in the link above for more details.
var paddedSamples = frameCount * 1152;
// ... we'll cover this below.
现在,我们已经有了样本总数,可以继续读出填充样本的数量。根据您使用的编码器,这可能被编写在嵌套在 Xing 标头中的 LAME 或 Lavf 标记下。此标头之后正好 17 个字节,有 3 个字节,分别以 12 位表示前端和末尾填充。
xingDataIndex = byteStr.indexOf('LAME');
if (xingDataIndex == -1) xingDataIndex = byteStr.indexOf('Lavf');
if (xingDataIndex != -1) {
// See http://gabriel.mp3-tech.org/mp3infotag.html#delays for details of
// how this information is encoded and parsed.
var gaplessDataIndex = xingDataIndex + 21;
var gaplessBits = ReadInt(byteStr.substr(gaplessDataIndex, 3));
// Upper 12 bits are the front padding, lower are the end padding.
frontPadding = gaplessBits >> 12;
endPadding = gaplessBits & 0xFFF;
}
realSamples = paddedSamples - (frontPadding + endPadding);
}
return {
audioDuration: realSamples * SECONDS_PER_SAMPLE,
frontPaddingDuration: frontPadding * SECONDS_PER_SAMPLE
};
}
至此,我们就有了一个完整的函数,可以解析绝大多数无间断内容。不过,极端情况无疑比比皆是,因此在生产环境中使用类似代码之前,建议谨慎行事。
附录 C:垃圾回收
系统会根据内容类型、平台特定限制和当前播放位置主动对属于 SourceBuffer
实例的内存进行垃圾回收。在 Chrome 中,首先会从已播放的缓冲区中回收内存。不过,如果内存用量超过平台特定限制,则会从未播放缓冲区中移除内存。
当播放因回收内存而到达时间轴上的某个间隙时,如果间隙过小,则可能会出现故障,如果间隙过大,则可能会出现完全停滞。这都不是很好的用户体验,因此请务必避免一次附加过多数据,并从媒体时间轴中手动移除不再需要的范围。
您可以通过对每个 SourceBuffer
使用 remove()
方法移除范围;其范围为 [start, end]
,以秒为单位。与 appendBuffer()
类似,每个 remove()
在完成后都会触发 updateend
事件。其他的移除或附加操作在事件触发之前不应发出。
在桌面版 Chrome 中,您可同时在内存中保存大约 12 MB 的音频内容和 150 MB 的视频内容。您不应跨浏览器或平台使用这些值;例如,它们绝不代表移动设备。
垃圾回收仅影响添加到 SourceBuffers
的数据;在 JavaScript 变量中缓冲的数据量没有限制。如有必要,您还可以在同一位置重新附加相同的数据。