Dual source blending
Combining two fragment shader outputs into a single framebuffer is called dual source blending. This technique is particularly useful for applications that require complex blending operations, such as those based on Porter-Duff blend modes. By replacing subsequent render passes with a single render pass, dual source blending can enhance performance and flexibility.
The new "dual-source-blending"
WebGPU feature lets you use the WGSL @blend_src
attribute at @location(0)
to denote the blending source index and the following blend factors: "src1"
, "one-minus-src1"
, "src1-alpha"
, and "one-minus-src1-alpha"
. See the following snippet, the chromestatus entry, and issue 341973423.
const adapter = await navigator.gpu.requestAdapter();
if (!adapter.features.has("dual-source-blending")) {
throw new Error("Dual source blending support is not available");
}
// Explicitly request dual source blending support.
const device = await adapter.requestDevice({
requiredFeatures: ["dual-source-blending"],
});
const code = `
enable dual_source_blending;
struct FragOut {
@location(0) @blend_src(0) color : vec4f,
@location(0) @blend_src(1) blend : vec4f,
}
@fragment fn main() -> FragOut {
var output : FragOut;
output.color = vec4f(1.0, 1.0, 1.0, 1.0);
output.blend = vec4f(0.5, 0.5, 0.5, 0.5);
return output;
}
`;
const shaderModule = device.createShaderModule({ code });
// Create a render pipeline with this shader module
// and run the shader on the GPU...
Shader compilation time improvements on Metal
The Chrome team is enhancing Tint, the WebGPU shader language compiler, by introducing an intermediate representation (IR) for devices that support WebGPU with the Metal backend. This IR, positioned between Tint's abstract syntax tree (AST) and the Metal backend writer, will make the compiler more efficient and maintainable, ultimately benefiting both developers and users. Initial tests show that the new version of Tint is up to 10 times faster when translating Unity's WGSL shaders to MSL.
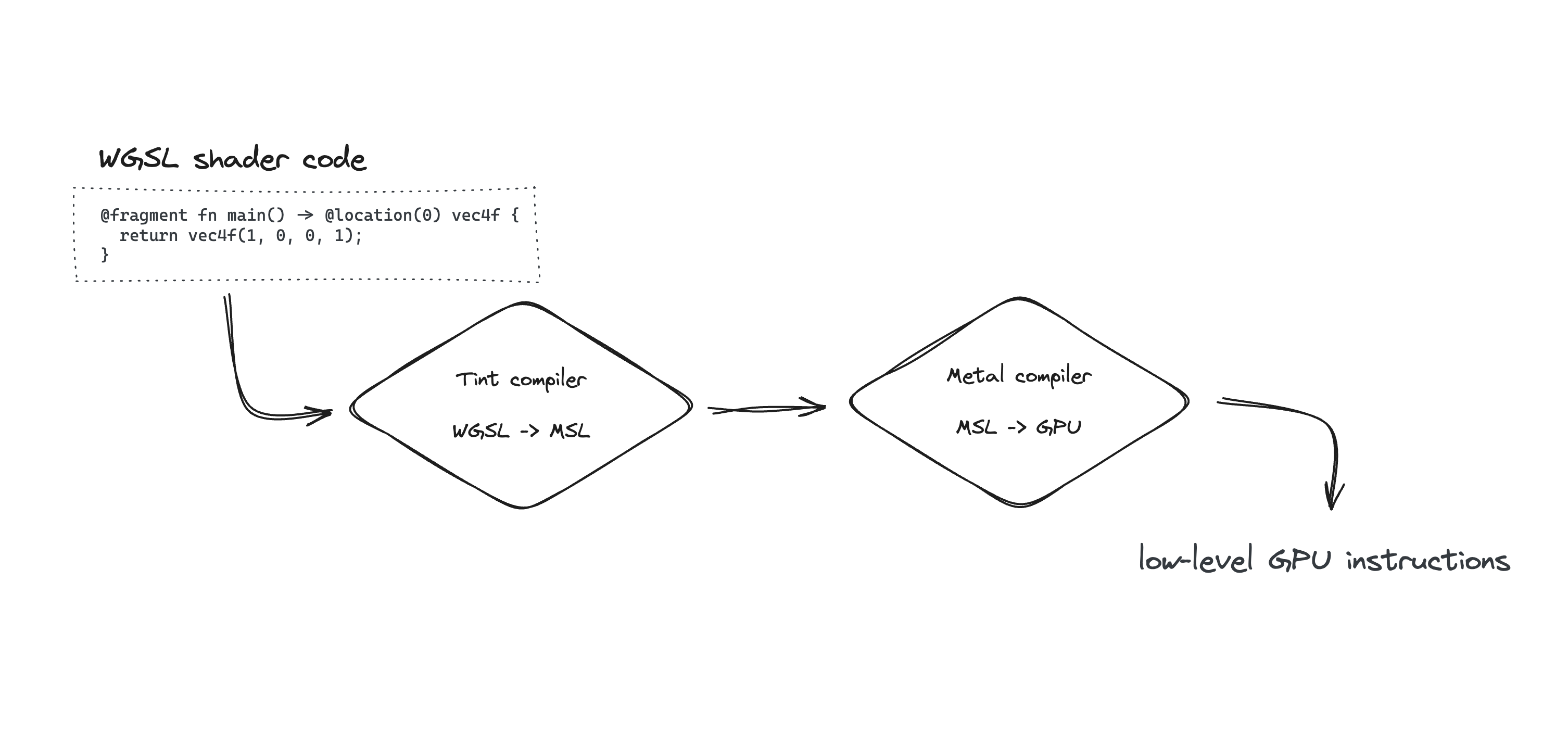
These improvements, already accessible on Android and ChromeOS, are being progressively expanded to macOS devices that support WebGPU with the Metal backend. See issue 42251016.
Deprecation of GPUAdapter requestAdapterInfo()
The GPUAdapter requestAdapterInfo()
asynchronous method is redundant as developers can already get GPUAdapterInfo synchronously using the GPUAdapter info
attribute. Hence, the non-standard GPUAdapter requestAdapterInfo()
method is now deprecated. See intent to deprecate.
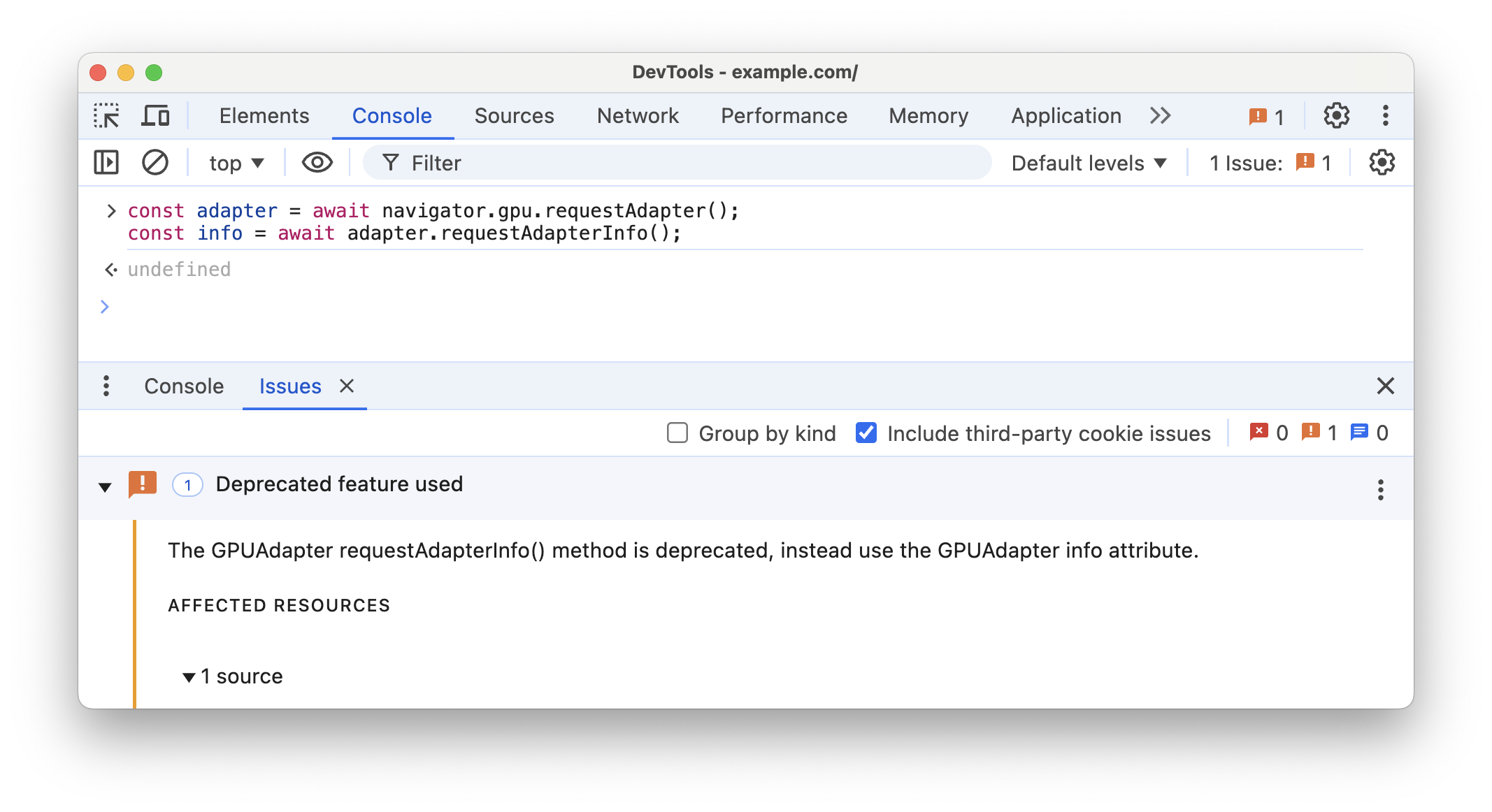
requestAdapterInfo()
in Chrome DevTools.Dawn updates
The webgpu.h C API has defined some naming conventions for extension structs. See the following name changes and issue 42241174.
WGPURenderPassDescriptor extensions
|
|
WGPURenderPassDescriptorMaxDrawCount ->
|
WGPURenderPassMaxDrawCount
|
WGPUShaderModuleDescriptor extensions
|
|
WGPUShaderModuleSPIRVDescriptor ->
|
WGPUShaderSourceSPIRV
|
WGPUShaderModuleWGSLDescriptor ->
|
WGPUShaderSourceWGSL
|
WGPUSurfaceDescriptor extensions
|
|
WGPUSurfaceDescriptorFromMetalLayer ->
|
WGPUSurfaceSourceMetalLayer
|
WGPUSurfaceDescriptorFromWindowsHWND ->
|
WGPUSurfaceSourceWindowsHWND
|
WGPUSurfaceDescriptorFromXlibWindow ->
|
WGPUSurfaceSourceXlibWindow
|
WGPUSurfaceDescriptorFromWaylandSurface ->
|
WGPUSurfaceSourceWaylandSurface
|
WGPUSurfaceDescriptorFromAndroidNativeWindow ->
|
WGPUSurfaceSourceAndroidNativeWindow
|
WGPUSurfaceDescriptorFromXcbWindow ->
|
WGPUSurfaceSourceXCBWindow
|
WGPUSurfaceDescriptorFromCanvasHTMLSelector ->
|
WGPUSurfaceSourceCanvasHTMLSelector_Emscripten
|
The WGPUDepthStencilState
's depthWriteEnabled
attribute type switches from boolean to WGPUOptionalBool
to better reflect its three possible states (true, false, and undefined) as in the JavaScript API. To learn more, see the following code snippet and the webgpu-headers PR.
wgpu::DepthStencilState depthStencilState = {};
depthStencilState.depthWriteEnabled = wgpu::OptionalBool::True; // Undefined by default
This covers only some of the key highlights. Check out the exhaustive list of commits.
What's New in WebGPU
A list of everything that has been covered in the What's New in WebGPU series.
Chrome 135
- Allow creating pipeline layout with null bind group layout
- Allow viewports to extend past the render targets bounds
- Easier access to the experimental compatibility mode on Android
- Remove maxInterStageShaderComponents limit
- Dawn updates
Chrome 134
- Improve machine-learning workloads with subgroups
- Remove float filterable texture types support as blendable
- Dawn updates
Chrome 133
- Additional unorm8x4-bgra and 1-component vertex formats
- Allow unknown limits to be requested with undefined value
- WGSL alignment rules changes
- WGSL performance gains with discard
- Use VideoFrame displaySize for external textures
- Handle images with non-default orientations using copyExternalImageToTexture
- Improving developer experience
- Enable compatibility mode with featureLevel
- Experimental subgroup features cleanup
- Deprecate maxInterStageShaderComponents limit
- Dawn updates
Chrome 132
- Texture view usage
- 32-bit float textures blending
- GPUDevice adapterInfo attribute
- Configuring canvas context with invalid format throw JavaScript error
- Filtering sampler restrictions on textures
- Extended subgroups experimentation
- Improving developer experience
- Experimental support for 16-bit normalized texture formats
- Dawn updates
Chrome 131
- Clip distances in WGSL
- GPUCanvasContext getConfiguration()
- Point and line primitives must not have depth bias
- Inclusive scan built-in functions for subgroups
- Experimental support for multi-draw indirect
- Shader module compilation option strict math
- Remove GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 130
- Dual source blending
- Shader compilation time improvements on Metal
- Deprecation of GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 129
Chrome 128
- Experimenting with subgroups
- Deprecate setting depth bias for lines and points
- Hide uncaptured error DevTools warning if preventDefault
- WGSL interpolate sampling first and either
- Dawn updates
Chrome 127
- Experimental support for OpenGL ES on Android
- GPUAdapter info attribute
- WebAssembly interop improvements
- Improved command encoder errors
- Dawn updates
Chrome 126
- Increase maxTextureArrayLayers limit
- Buffer upload optimization for Vulkan backend
- Shader compilation time improvements
- Submitted command buffers must be unique
- Dawn updates
Chrome 125
Chrome 124
- Read-only and read-write storage textures
- Service workers and shared workers support
- New adapter information attributes
- Bug fixes
- Dawn updates
Chrome 123
- DP4a built-in functions support in WGSL
- Unrestricted pointer parameters in WGSL
- Syntax sugar for dereferencing composites in WGSL
- Separate read-only state for stencil and depth aspects
- Dawn updates
Chrome 122
- Expand reach with compatibility mode (feature in development)
- Increase maxVertexAttributes limit
- Dawn updates
Chrome 121
- Support WebGPU on Android
- Use DXC instead of FXC for shader compilation on Windows
- Timestamp queries in compute and render passes
- Default entry points to shader modules
- Support display-p3 as GPUExternalTexture color space
- Memory heaps info
- Dawn updates
Chrome 120
- Support for 16-bit floating-point values in WGSL
- Push the limits
- Changes to depth-stencil state
- Adapter information updates
- Timestamp queries quantization
- Spring-cleaning features
Chrome 119
- Filterable 32-bit float textures
- unorm10-10-10-2 vertex format
- rgb10a2uint texture format
- Dawn updates
Chrome 118
- HTMLImageElement and ImageData support in
copyExternalImageToTexture()
- Experimental support for read-write and read-only storage texture
- Dawn updates
Chrome 117
- Unset vertex buffer
- Unset bind group
- Silence errors from async pipeline creation when device is lost
- SPIR-V shader module creation updates
- Improving developer experience
- Caching pipelines with automatically generated layout
- Dawn updates
Chrome 116
- WebCodecs integration
- Lost device returned by GPUAdapter
requestDevice()
- Keep video playback smooth if
importExternalTexture()
is called - Spec conformance
- Improving developer experience
- Dawn updates
Chrome 115
- Supported WGSL language extensions
- Experimental support for Direct3D 11
- Get discrete GPU by default on AC power
- Improving developer experience
- Dawn updates
Chrome 114
- Optimize JavaScript
- getCurrentTexture() on unconfigured canvas throws InvalidStateError
- WGSL updates
- Dawn updates