Supported WGSL language extensions
The wgslLanguageFeatures
member of the GPU
object lists the names of supported WGSL language extensions. Supported WGSL language extensions are automatically enabled, therefore you don’t need to explicitly request one. This list is currently empty but you can expect plenty of them in the future (for example, do-while loops
). See issue dawn:1777.
if (navigator.gpu.wgslLanguageFeatures?.has("unknown-feature")) {
// Use unknown-feature in WGSL shader code.
}
Experimental support for Direct3D 11
The Chromium team is working on adding WebGPU support for Direct3D 11. You can now experiment with it locally by running Chrome on Windows with the --enable-unsafe-webgpu --use-webgpu-adapter=d3d11
command-line flags. See issue dawn:1705.
Get discrete GPU by default on AC power
On dual GPU macOS devices, if requestAdapter()
is called without a powerPreference
option, the discrete GPU is returned when the user’s device is on AC power. Otherwise, the integrated GPU is returned. See change 4499307.
Improving developer experience
New DevTools warnings
If the depth
key is used in a GPUExtend3DDict
a warning is shown in the DevTools Console since the correct key is depthOrArrayLayers
. See issue chromium:1440900.
A warning is also raised if a GPUBlendComponent
has a mix of explicit and defaulted members. See issue dawn:1785.
Even though zero-size dispatches and draws are valid, a warning encourages developers to avoid them when possible. See issue dawn:1786.
Better error messages
An improved error message is now provided when using a GPUCommandEncoder
if finish()
has been called already. See issue dawn:1736.
When submitting command buffers with destroyed objects, the labels of the command buffers that were used in submit()
are now visible in the error message. See issue dawn:1747.
The invalid part of the depth stencil state is now specified in the error message when validating depthStencil
. See issue dawn:1735.
The minBindingSize
validation error message now reports the group and number of the binding that failed validation, as well as the buffer. See issue dawn:1604.
Error messages returned by the mapAsync()
method on a GPUBuffer
object have been improved to help developers when debugging. See an example below and issue chromium:1431622.
// Create a GPU buffer and map it.
const descriptor = { size: 0, usage: GPUBufferUsage.MAP_READ };
const buffer = device.createBuffer(descriptor);
buffer.mapAsync(GPUMapMode.READ);
// Before it has been mapped, request another mapping.
try {
await buffer.mapAsync(GPUMapMode.READ);
} catch (error) {
// New! Error message tells you mapping is already pending.
console.warn(error.message);
}
Labels in macOS debugging tools
The use_user_defined_labels_in_backend
debug toggle allows you to forward object labels to the backend so that they can be seen in platform-specific debugging tools like RenderDoc, PIX, or Instruments. From now on, a better debug experience is provided on macOS when you enable it for debugging. See issue dawn:1784
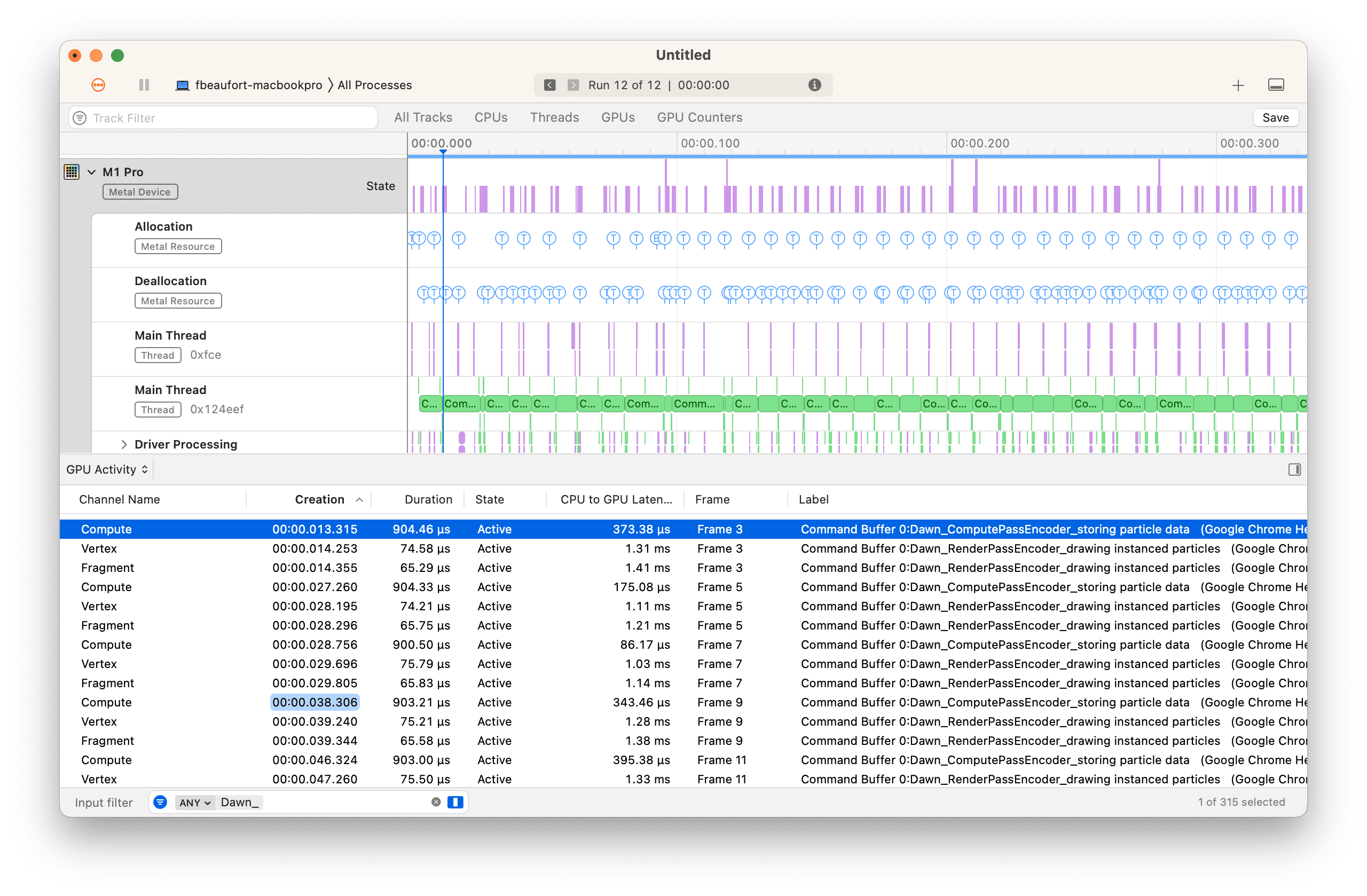
Log HLSL if compilation fails
The dump_shaders
debug toggle allows you to log input WGSL shaders and translated backend shaders. From now on, when you enable it for debugging, the HLSL will be dumped if it fails compilation. See issue dawn:1681
Dawn updates
Unset vertex buffer
Passing nullptr
rather than a wgpu::Buffer
to SetVertexBuffer()
on wgpu::RenderPassEncoder
or wgpu::RenderBundleEncoder
allows you to unset a previously set vertex buffer in a given slot. See issue dawn:1675.
// Set vertex buffer in slot 0.
myRenderPassEncoder.SetVertexBuffer(0, myVertexBuffer);
// Then later, unset vertex buffer in slot 0.
myRenderPassEncoder.SetVertexBuffer(0, nullptr);
Transient attachments
You can create attachments that allow render pass operations to stay in tile memory, avoiding VRAM traffic and potentially avoiding VRAM allocation for the textures by setting the wgpu::TextureUsage::TransientAttachment
usage. This feature is supported only for Metal and Vulkan. See issue dawn: 1695.
wgpu::TextureDescriptor desc;
desc.format = wgpu::TextureFormat::RGBA8Unorm;
desc.size = {1, 1, 1};
desc.usage = wgpu::TextureUsage::RenderAttachment |
wgpu::TextureUsage::TransientAttachment;
auto transientTexture = device.CreateTexture(&desc);
// You can now create views from the texture to serve as transient
// attachments, e.g. as color attachments in a render pipeline.
Building without depot_tools
A new DAWN_FETCH_DEPENDENCIES
CMake option allows you to fetch Dawn dependencies using a Python script that reads DEPS files instead of requiring the installation of depot_tools
by all projects that depend on it. See change 131750.
What's New in WebGPU
A list of everything that has been covered in the What's New in WebGPU series.
Chrome 134
- Improve machine-learning workloads with subgroups
- Remove float filterable texture types support as blendable
- Dawn updates
Chrome 133
- Additional unorm8x4-bgra and 1-component vertex formats
- Allow unknown limits to be requested with undefined value
- WGSL alignment rules changes
- WGSL performance gains with discard
- Use VideoFrame displaySize for external textures
- Handle images with non-default orientations using copyExternalImageToTexture
- Improving developer experience
- Enable compatibility mode with featureLevel
- Experimental subgroup features cleanup
- Deprecate maxInterStageShaderComponents limit
- Dawn updates
Chrome 132
- Texture view usage
- 32-bit float textures blending
- GPUDevice adapterInfo attribute
- Configuring canvas context with invalid format throw JavaScript error
- Filtering sampler restrictions on textures
- Extended subgroups experimentation
- Improving developer experience
- Experimental support for 16-bit normalized texture formats
- Dawn updates
Chrome 131
- Clip distances in WGSL
- GPUCanvasContext getConfiguration()
- Point and line primitives must not have depth bias
- Inclusive scan built-in functions for subgroups
- Experimental support for multi-draw indirect
- Shader module compilation option strict math
- Remove GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 130
- Dual source blending
- Shader compilation time improvements on Metal
- Deprecation of GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 129
Chrome 128
- Experimenting with subgroups
- Deprecate setting depth bias for lines and points
- Hide uncaptured error DevTools warning if preventDefault
- WGSL interpolate sampling first and either
- Dawn updates
Chrome 127
- Experimental support for OpenGL ES on Android
- GPUAdapter info attribute
- WebAssembly interop improvements
- Improved command encoder errors
- Dawn updates
Chrome 126
- Increase maxTextureArrayLayers limit
- Buffer upload optimization for Vulkan backend
- Shader compilation time improvements
- Submitted command buffers must be unique
- Dawn updates
Chrome 125
Chrome 124
- Read-only and read-write storage textures
- Service workers and shared workers support
- New adapter information attributes
- Bug fixes
- Dawn updates
Chrome 123
- DP4a built-in functions support in WGSL
- Unrestricted pointer parameters in WGSL
- Syntax sugar for dereferencing composites in WGSL
- Separate read-only state for stencil and depth aspects
- Dawn updates
Chrome 122
- Expand reach with compatibility mode (feature in development)
- Increase maxVertexAttributes limit
- Dawn updates
Chrome 121
- Support WebGPU on Android
- Use DXC instead of FXC for shader compilation on Windows
- Timestamp queries in compute and render passes
- Default entry points to shader modules
- Support display-p3 as GPUExternalTexture color space
- Memory heaps info
- Dawn updates
Chrome 120
- Support for 16-bit floating-point values in WGSL
- Push the limits
- Changes to depth-stencil state
- Adapter information updates
- Timestamp queries quantization
- Spring-cleaning features
Chrome 119
- Filterable 32-bit float textures
- unorm10-10-10-2 vertex format
- rgb10a2uint texture format
- Dawn updates
Chrome 118
- HTMLImageElement and ImageData support in
copyExternalImageToTexture()
- Experimental support for read-write and read-only storage texture
- Dawn updates
Chrome 117
- Unset vertex buffer
- Unset bind group
- Silence errors from async pipeline creation when device is lost
- SPIR-V shader module creation updates
- Improving developer experience
- Caching pipelines with automatically generated layout
- Dawn updates
Chrome 116
- WebCodecs integration
- Lost device returned by GPUAdapter
requestDevice()
- Keep video playback smooth if
importExternalTexture()
is called - Spec conformance
- Improving developer experience
- Dawn updates
Chrome 115
- Supported WGSL language extensions
- Experimental support for Direct3D 11
- Get discrete GPU by default on AC power
- Improving developer experience
- Dawn updates
Chrome 114
- Optimize JavaScript
- getCurrentTexture() on unconfigured canvas throws InvalidStateError
- WGSL updates
- Dawn updates