Read-only and read-write storage textures
The storage texture binding type allows shaders to read from storage textures without adding the TEXTURE_BINDING
usage, and perform mixed reads and writes on certain formats. When the "readonly_and_readwrite_storage_textures"
WGSL language extension is present in navigator.gpu.wgslLanguageFeatures
, you can now set GPUStorageTexture
access to either "read-write"
or "read-only"
when creating a bind group layout. Previously this was restricted to "write-only"
.
Then, your WGSL shader code can use read_write
and read
access qualifier for storage textures, the textureLoad()
and textureStore()
built-in functions behave accordingly, and a new textureBarrier()
built-in function is available to synchronize texture memory accesses in a workgroup.
It's recommended to use a requires-directive to signal the potential for non-portability with requires readonly_and_readwrite_storage_textures;
at the top of your WGSL shader code. See the following example and issue dawn:1972.
if (!navigator.gpu.wgslLanguageFeatures.has("readonly_and_readwrite_storage_textures")) {
throw new Error("Read-only and read-write storage textures are not available");
}
const adapter = await navigator.gpu.requestAdapter();
const device = await adapter.requestDevice();
const bindGroupLayout = device.createBindGroupLayout({
entries: [{
binding: 0,
visibility: GPUShaderStage.COMPUTE,
storageTexture: {
access: "read-write", // <-- New!
format: "r32uint",
},
}],
});
const shaderModule = device.createShaderModule({ code: `
requires readonly_and_readwrite_storage_textures;
@group(0) @binding(0) var tex : texture_storage_2d<r32uint, read_write>;
@compute @workgroup_size(1, 1)
fn main(@builtin(local_invocation_id) local_id: vec3u) {
var data = textureLoad(tex, vec2i(local_id.xy));
data.x *= 2;
textureStore(tex, vec2i(local_id.xy), data);
}`
});
// You can now create a compute pipeline with this shader module and
// send the appropriate commands to the GPU.
Service workers and shared workers support
WebGPU in Chrome takes web workers support to the next level, now offering support for both service workers and shared workers. You can use service workers to enhance background tasks and offline capabilities, and shared workers for efficient resource sharing across scripts. See issue chromium:41494731.
Check out the chrome extension sample and WebLLM chrome extension to see how to use WebGPU in an extension service worker.
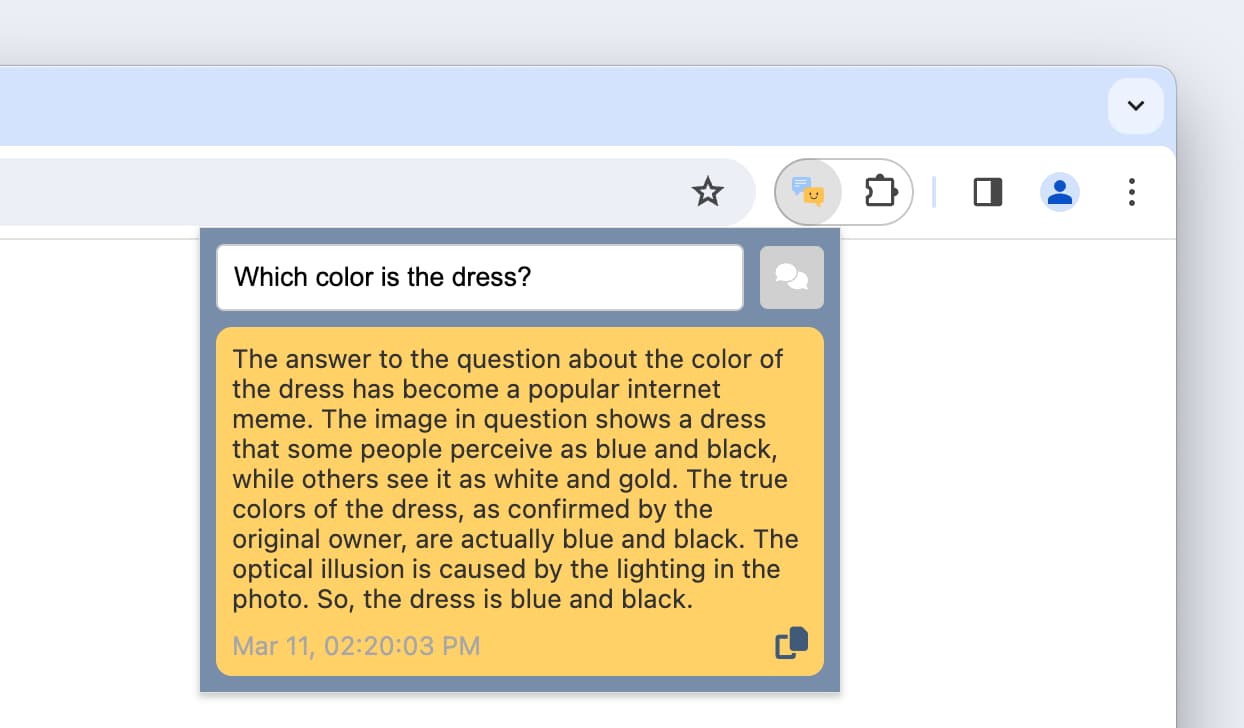
New adapter information attributes
Non-standard d3dShaderModel
and vkDriverVersion
adapter info attributes are now available upon calling requestAdapterInfo()
if the user has enabled the "WebGPU Developer Features" flag at chrome://flags/#enable-webgpu-developer-features
. When supported:
The
d3dShaderModel
is the maximum supported D3D shader model number. For example, the value 62 indicates that the current driver supports HLSL SM 6.2. See documentation and issue dawn:1254.The
vkDriverVersion
is the vendor-specified version number of the Vulkan driver. See documentation and issue chromium:327457605.
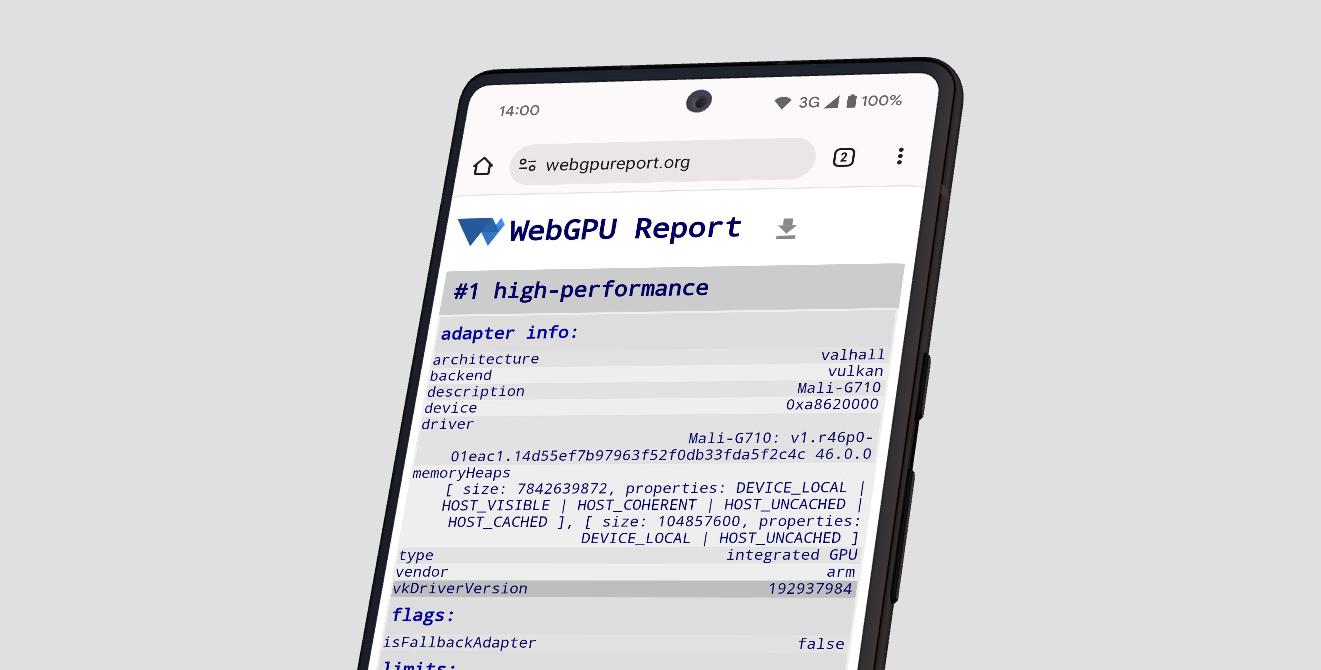
vkDriverVersion
shown on https://webgpureport.org.Bug fixes
Creating two pipelines with matching bindgroups using layout: "auto"
, then creating a bindgroup with the first pipeline, and using it on the second pipeline now raises a GPUValidationError. Allowing it was an implementation bug which is now fixed with proper tests. See issue dawn:2402.
Dawn updates
In the Dawn API, the uncaptured error callback set with wgpuDeviceSetUncapturedErrorCallback
is now not called after the GPU device is lost. This fix aligns Dawn with the JavaScript API specification and Blink's implementation. See issue dawn:2459.
This covers only some of the key highlights. Check out the exhaustive list of commits.
What's New in WebGPU
A list of everything that has been covered in the What's New in WebGPU series.
Chrome 131
- Clip distances in WGSL
- GPUCanvasContext getConfiguration()
- Point and line primitives must not have depth bias
- Inclusive scan built-in functions for subgroups
- Experimental support for multi-draw indirect
- Shader module compilation option strict math
- Remove GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 130
- Dual source blending
- Shader compilation time improvements on Metal
- Deprecation of GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 129
Chrome 128
- Experimenting with subgroups
- Deprecate setting depth bias for lines and points
- Hide uncaptured error DevTools warning if preventDefault
- WGSL interpolate sampling first and either
- Dawn updates
Chrome 127
- Experimental support for OpenGL ES on Android
- GPUAdapter info attribute
- WebAssembly interop improvements
- Improved command encoder errors
- Dawn updates
Chrome 126
- Increase maxTextureArrayLayers limit
- Buffer upload optimization for Vulkan backend
- Shader compilation time improvements
- Submitted command buffers must be unique
- Dawn updates
Chrome 125
Chrome 124
- Read-only and read-write storage textures
- Service workers and shared workers support
- New adapter information attributes
- Bug fixes
- Dawn updates
Chrome 123
- DP4a built-in functions support in WGSL
- Unrestricted pointer parameters in WGSL
- Syntax sugar for dereferencing composites in WGSL
- Separate read-only state for stencil and depth aspects
- Dawn updates
Chrome 122
- Expand reach with compatibility mode (feature in development)
- Increase maxVertexAttributes limit
- Dawn updates
Chrome 121
- Support WebGPU on Android
- Use DXC instead of FXC for shader compilation on Windows
- Timestamp queries in compute and render passes
- Default entry points to shader modules
- Support display-p3 as GPUExternalTexture color space
- Memory heaps info
- Dawn updates
Chrome 120
- Support for 16-bit floating-point values in WGSL
- Push the limits
- Changes to depth-stencil state
- Adapter information updates
- Timestamp queries quantization
- Spring-cleaning features
Chrome 119
- Filterable 32-bit float textures
- unorm10-10-10-2 vertex format
- rgb10a2uint texture format
- Dawn updates
Chrome 118
- HTMLImageElement and ImageData support in
copyExternalImageToTexture()
- Experimental support for read-write and read-only storage texture
- Dawn updates
Chrome 117
- Unset vertex buffer
- Unset bind group
- Silence errors from async pipeline creation when device is lost
- SPIR-V shader module creation updates
- Improving developer experience
- Caching pipelines with automatically generated layout
- Dawn updates
Chrome 116
- WebCodecs integration
- Lost device returned by GPUAdapter
requestDevice()
- Keep video playback smooth if
importExternalTexture()
is called - Spec conformance
- Improving developer experience
- Dawn updates
Chrome 115
- Supported WGSL language extensions
- Experimental support for Direct3D 11
- Get discrete GPU by default on AC power
- Improving developer experience
- Dawn updates
Chrome 114
- Optimize JavaScript
- getCurrentTexture() on unconfigured canvas throws InvalidStateError
- WGSL updates
- Dawn updates