WebCodecs integration
WebGPU exposes an API to create opaque "external texture" objects from HTMLVideoElement
through importExternalTexture()
. You can use these objects to sample the video frames efficiently, potentially in a 0-copy way directly from the source YUV color model data.
However, the initial WebGPU specification did not allow creating GPUExternalTexture
objects from WebCodecs VideoFrame
objects. This capability is important for advanced video processing apps that already use WebCodecs and would like to integrate WebGPU in the video processing pipeline. WebCodecs integration adds support for using a VideoFrame
as the source for a GPUExternalTexture
and a copyExternalImageToTexture()
call. See the following example, and the chromestatus entry.
// Access the GPU device.
const adapter = await navigator.gpu.requestAdapter();
const device = await adapter.requestDevice();
// Create VideoFrame from HTMLVideoElement.
const video = document.querySelector("video");
const videoFrame = new VideoFrame(video);
// Create texture from VideoFrame.
const texture = device.importExternalTexture({ source: videoFrame });
// TODO: Use texture in bind group creation.
Check out the Video Uploading with WebCodecs experimental sample to play with it.
Lost device returned by GPUAdapter requestDevice()
If the requestDevice()
method on GPUAdapter
fails because it has been already used to create a GPUDevice
, it now fulfills with a GPUDevice
immediately marked as lost, rather than returning a promise that rejects with null
. See issue chromium:1234617.
const adapter = await navigator.gpu.requestAdapter();
const device1 = await adapter.requestDevice();
// New! The promise is not rejected anymore with null.
const device2 = await adapter.requestDevice();
// And the device is immediately marked as lost.
const info = await device2.lost;
Keep video playback smooth if importExternalTexture() is called
When importExternalTexture()
is called with an HTMLVideoElement
, the associated video playback is not throttled anymore when the video is not visible in the viewport. See issue chromium:1425252.
Spec conformance
The message
argument in the GPUPipelineError()
constructor is optional. See change chromium:4613967.
An error is fired when calling createShaderModule()
if the WGSL source code
contains contains \0
. See issue dawn:1345.
The default maximum level of detail (lodMaxClamp
) used when sampling a texture with createSampler()
is 32. See change chromium:4608063.
Improving developer experience
A message is displayed in the DevTools JavaScript console to remind developers when they are using WebGPU on an unsupported platform. See change chromium:4589369.
Buffer validation error messages are instantly shown in DevTools JavaScript console when getMappedRange()
fails without forcing developers to send commands to the queue. See change chromium:4597950.
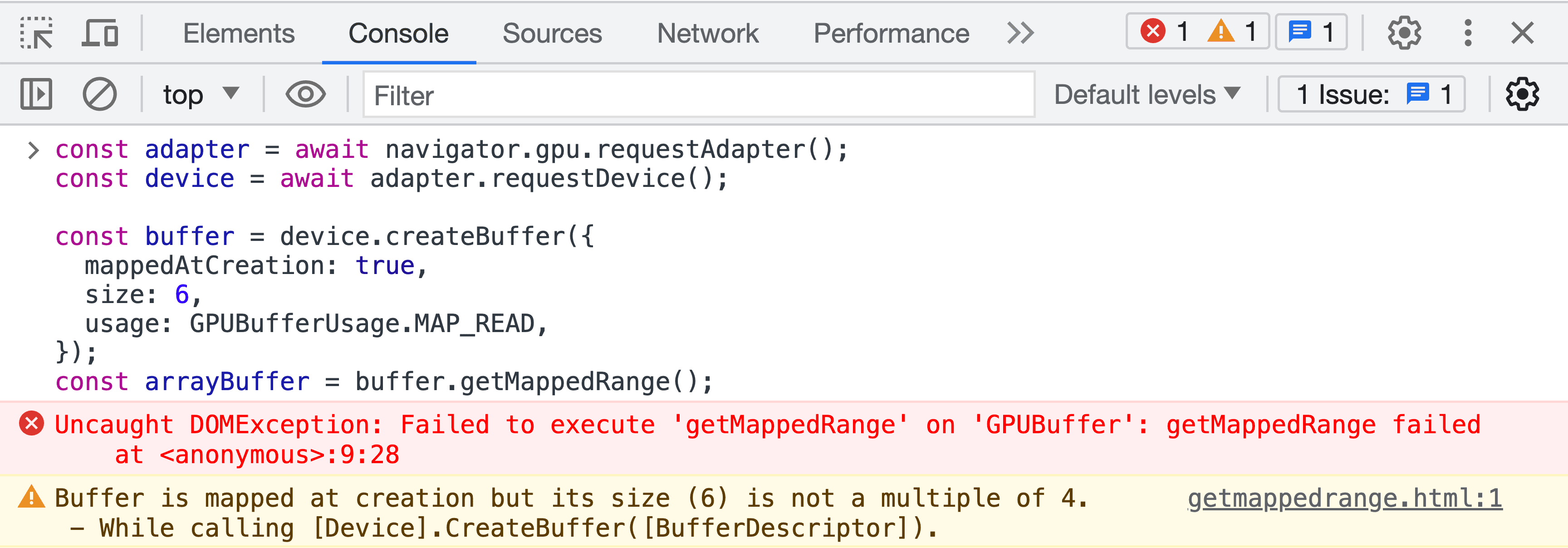
Dawn updates
The disallow_unsafe_apis
debug toggle has been renamed to allow_unsafe_apis
and made its default to disabled. This toggle suppresses validation errors on API entry points or parameter combinations that aren't considered secure yet. It can be useful for debugging.
See issue dawn:1685.
The wgpu::ShaderModuleWGSLDescriptor
deprecated source
attribute is removed in favor of code
. See change dawn:130321.
The missing wgpu::RenderBundle::SetLabel()
method has been implemented. See change dawn:134502.
Applications can request a particular backend when getting an adapter with the wgpu::RequestAdapterOptionsBackendType
option. See an example below and issue dawn:1875.
wgpu::RequestAdapterOptionsBackendType backendTypeOptions = {};
backendTypeOptions.backendType = wgpu::BackendType::D3D12;
wgpu::RequestAdapterOptions options = {};
options.nextInChain = &backendTypeOptions;
// Request D3D12 adapter.
myInstance.RequestAdapter(&options, myCallback, myUserData);
A new SwapChain::GetCurrentTexture()
method has been added with additional usages for swapchain textures so that the return wgpu::Texture
can be used in copies. See an example below and issue dawn:1551.
wgpu::SwapChain swapchain = myDevice.CreateSwapChain(mySurface, &myDesc);
swapchain.GetCurrentTexture();
swapchain.Present();
This covers only some of the key highlights. Check out the exhaustive list of commits.
What's New in WebGPU
A list of everything that has been covered in the What's New in WebGPU series.
Chrome 133
- Additional unorm8x4-bgra and 1-component vertex formats
- Allow unknown limits to be requested with undefined value
- WGSL alignment rules changes
- WGSL performance gains with discard
- Use VideoFrame displaySize for external textures
- Handle images with non-default orientations using copyExternalImageToTexture
- Improving developer experience
- Enable compatibility mode with featureLevel
- Experimental subgroup features cleanup
- Deprecate maxInterStageShaderComponents limit
- Dawn updates
Chrome 132
- Texture view usage
- 32-bit float textures blending
- GPUDevice adapterInfo attribute
- Configuring canvas context with invalid format throw JavaScript error
- Filtering sampler restrictions on textures
- Extended subgroups experimentation
- Improving developer experience
- Experimental support for 16-bit normalized texture formats
- Dawn updates
Chrome 131
- Clip distances in WGSL
- GPUCanvasContext getConfiguration()
- Point and line primitives must not have depth bias
- Inclusive scan built-in functions for subgroups
- Experimental support for multi-draw indirect
- Shader module compilation option strict math
- Remove GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 130
- Dual source blending
- Shader compilation time improvements on Metal
- Deprecation of GPUAdapter requestAdapterInfo()
- Dawn updates
Chrome 129
Chrome 128
- Experimenting with subgroups
- Deprecate setting depth bias for lines and points
- Hide uncaptured error DevTools warning if preventDefault
- WGSL interpolate sampling first and either
- Dawn updates
Chrome 127
- Experimental support for OpenGL ES on Android
- GPUAdapter info attribute
- WebAssembly interop improvements
- Improved command encoder errors
- Dawn updates
Chrome 126
- Increase maxTextureArrayLayers limit
- Buffer upload optimization for Vulkan backend
- Shader compilation time improvements
- Submitted command buffers must be unique
- Dawn updates
Chrome 125
Chrome 124
- Read-only and read-write storage textures
- Service workers and shared workers support
- New adapter information attributes
- Bug fixes
- Dawn updates
Chrome 123
- DP4a built-in functions support in WGSL
- Unrestricted pointer parameters in WGSL
- Syntax sugar for dereferencing composites in WGSL
- Separate read-only state for stencil and depth aspects
- Dawn updates
Chrome 122
- Expand reach with compatibility mode (feature in development)
- Increase maxVertexAttributes limit
- Dawn updates
Chrome 121
- Support WebGPU on Android
- Use DXC instead of FXC for shader compilation on Windows
- Timestamp queries in compute and render passes
- Default entry points to shader modules
- Support display-p3 as GPUExternalTexture color space
- Memory heaps info
- Dawn updates
Chrome 120
- Support for 16-bit floating-point values in WGSL
- Push the limits
- Changes to depth-stencil state
- Adapter information updates
- Timestamp queries quantization
- Spring-cleaning features
Chrome 119
- Filterable 32-bit float textures
- unorm10-10-10-2 vertex format
- rgb10a2uint texture format
- Dawn updates
Chrome 118
- HTMLImageElement and ImageData support in
copyExternalImageToTexture()
- Experimental support for read-write and read-only storage texture
- Dawn updates
Chrome 117
- Unset vertex buffer
- Unset bind group
- Silence errors from async pipeline creation when device is lost
- SPIR-V shader module creation updates
- Improving developer experience
- Caching pipelines with automatically generated layout
- Dawn updates
Chrome 116
- WebCodecs integration
- Lost device returned by GPUAdapter
requestDevice()
- Keep video playback smooth if
importExternalTexture()
is called - Spec conformance
- Improving developer experience
- Dawn updates
Chrome 115
- Supported WGSL language extensions
- Experimental support for Direct3D 11
- Get discrete GPU by default on AC power
- Improving developer experience
- Dawn updates
Chrome 114
- Optimize JavaScript
- getCurrentTexture() on unconfigured canvas throws InvalidStateError
- WGSL updates
- Dawn updates